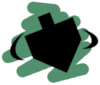
BlackTopp Studios
inc
Go to the documentation of this file.
40 #ifndef _crossplatformexports_h
41 #define _crossplatformexports_h
106 #endif // MEZZ_WINDOWS
107 #endif // \NONWINDOWS
115 #define _MEZZ_THREAD_WIN32_
118 #pragma warning( disable : 4251) // Disable the dll import/export warnings on items that
120 #pragma warning( disable : 4244) // Disable the double to float conversions, they are in
123 #pragma warning( disable : 4127) // Disable conditional expression is constant
124 #pragma warning( disable : 4800) // pugi xml unspecified bool type coercion has
126 #pragma warning( disable : 4221) // interfaces don't generate linkable symbols, well of
128 #pragma warning( disable : 4512) // Could not generate assignment operator for class
133 #ifdef EXPORTINGMEZZANINEDLL
134 #define MEZZ_LIB __declspec(dllexport)
136 #define MEZZ_LIB __declspec(dllimport)
137 #endif // \EXPORTINGMEZZANINEDLL
144 #define _MEZZ_THREAD_POSIX_
145 #if defined(__APPLE__) || defined(__MACH__) || defined(__OSX__)
149 #define _MEZZ_THREAD_APPLE_
153 #define _MEZZ_PLATFORM_DEFINED_
158 #ifndef MEZZ_DEPRECATED
159 #if defined(__GNUC__)
160 #define MEZZ_DEPRECATED __attribute__((deprecated))
161 #elif defined(_MSC_VER) && _MSC_VER >= 1300
162 #define MEZZ_DEPRECATED __declspec(deprecated)
164 #define MEZZ_DEPRECATED
181 #ifndef MEZZ_USEATOMICSTODECACHECOMPLETEWORK
182 #define MEZZ_USEATOMICSTODECACHECOMPLETEWORK
184 #ifndef _MEZZ_DECACHEWORKUNIT_
185 #undef MEZZ_USEATOMICSTODECACHECOMPLETEWORK
191 #ifndef MEZZ_FRAMESTOTRACK
192 #define MEZZ_FRAMESTOTRACK 10
194 #ifdef _MEZZ_FRAMESTOTRACK_
195 #undef MEZZ_FRAMESTOTRACK
196 #define MEZZ_FRAMESTOTRACK _MEZZ_FRAMESTOTRACK_
203 #define WINAPI __stdcall
205 #define WINAPI ErrorThisOnlyGoesInwin32Code
229 #ifdef _MSC_VER // The intel compiler might act up here
230 #define SAVE_WARNING_STATE PRAGMA(warning(push))
231 #define SUPPRESS_VC_WARNING(suppress) PRAGMA(warning(disable: ## suppress ## ))
232 #define SUPPRESS_GCC_WARNING(suppress)
233 #define RESTORE_WARNING_STATE PRAGMA(warning(pop))
234 #define PRAGMA(x) __pragma(x)
236 #define SAVE_WARNING_STATE PRAGMA(GCC diagnostic push)
237 #define SUPPRESS_VC_WARNING(X)
238 #define SUPPRESS_GCC_WARNING(X) PRAGMA(GCC diagnostic ignored X)
239 #define RESTORE_WARNING_STATE PRAGMA(GCC diagnostic pop)
240 #define PRAGMA(x) _Pragma(#x)